Package in Java
package MyPack;
public class Balance{
String name;
double bal;
public Balance(String n, double b){
this.name=n;
this.bal=b;
}
public void show(){
if(bal<0)
System.out.print("LESS THAN ZERO BALANCE -->");
System.out.println(name +": $" +bal);
}
}
-------------------------------------------------------------------------------------------------------------------
import MyPack.Balance;
class AccountBalance{
public static void main(String args[]){
Balance current[] = new Balance[3];
current[0] = new Balance("SAIKUMAR S", 502.53);
current[1] = new Balance("RAJESH M", 268.06);
current[2] = new Balance("MURALI S ", -12.33);
for (int i=0;i<3;i++)
current[i].show();
}
}//For Compilation javac -d . Balance.java (because package)
// A simple package
package MyPack;
public class Balance{
String name;
double bal;
public Balance(String n, double b){
this.name=n;
this.bal=b;
}
public void show(){
if(bal<0)
System.out.print("LESS THAN ZERO BALANCE -->");
System.out.println(name +": $" +bal);
}
}
-------------------------------------------------------------------------------------------------------------------
import MyPack.Balance;
class AccountBalance{
public static void main(String args[]){
Balance current[] = new Balance[3];
current[0] = new Balance("SAIKUMAR S", 502.53);
current[1] = new Balance("RAJESH M", 268.06);
current[2] = new Balance("MURALI S ", -12.33);
for (int i=0;i<3;i++)
current[i].show();
}
}//For Compilation javac -d . Balance.java (because package)
javac AccountBalance.java
java AccountBalance
class staticdemo{
int area(int i){
return i*i;
}
int area(int a, int b){
return a*b;
}
}
public class MethodOverloading {
public static void main(String[] args) {
Test t = new Test();
int area;
area = t.area(5);
System.err.println("Area of a square is :"+area);
area = t.area(5,6);
System.out.println("Area of a rectangle is :"+area);
}
}
Static members in java
class staticdemo{
int objno;
static int objcnt;
void hitcount(){
objno = ++objcnt;
}
void showhits(){
System.out.println("This page has been hit for :"+objcnt);
}
void showposition(){
System.out.println("client"+objno +"/" +objcnt);
}
}
public class Static {
public static void main(String[] args) {
staticdemo s = new staticdemo();
staticdemo t = new staticdemo();
staticdemo v = new staticdemo();
s.hitcount();
t.hitcount();
v.hitcount();
v.showhits();
s.showposition();
t.showposition();
v.showposition();
}
}
Method Overloading in java program
class Test{int area(int i){
return i*i;
}
int area(int a, int b){
return a*b;
}
}
public class MethodOverloading {
public static void main(String[] args) {
Test t = new Test();
int area;
area = t.area(5);
System.err.println("Area of a square is :"+area);
area = t.area(5,6);
System.out.println("Area of a rectangle is :"+area);
}
}
Multiple if else statement using java(if stmt else condition)
import java.util.*;public class Condition {
public static void main(String[] args) {
int a[]= new int[1] ;
Scanner sc=new Scanner(System.in);
System.out.println("Please enter elements between 0 and 6");
for(int j=0;j<1;j++)
a[j] = sc.nextInt();
for(int j=0;j<1;j++)
if(a[j]==0)
{
System.out.println("Sunday!");
break;}
else if(a[j]==1)
{
System.out.println("Monday!");
break;}
else if(a[j]==2)
{
System.out.println("Tuesday!");
break;}
else if(a[j]==3)
{
System.out.println("Wednesday!");
break;}
else if(a[j]==4)
{
System.out.println("Thursday!");
break; }
else if(a[j]==5)
{
System.out.println("Friday!");
break;}
else if(a[j]==6)
{
System.out.println("Saturday!");
break;}
else
{
System.out.println("Sorry Invallid option! Enter the number between the range 0 to 6");
break; }
}
}
Java button applet program
import java.awt.*;
public class ButtonActionsTest extends java.applet.Applet {
public void init() {
setBackground(Color.white);
add(new Button("Red"));
add(new Button("Blue"));
add(new Button("Green"));
add(new Button("White"));
add(new Button("Black"));
}
public boolean action(Event evt, Object arg) {
if (evt.target instanceof Button)
changeColor((String)arg);
return true;
}
void changeColor(String bname) {
if (bname.equals("Red")) setBackground(Color.red);
else if (bname.equals("Blue")) setBackground(Color.blue);
else if (bname.equals("Green")) setBackground(Color.green);
else if (bname.equals("White")) setBackground(Color.white);
else setBackground(Color.black);
}
}
public class ButtonActionsTest extends java.applet.Applet {
public void init() {
setBackground(Color.white);
add(new Button("Red"));
add(new Button("Blue"));
add(new Button("Green"));
add(new Button("White"));
add(new Button("Black"));
}
public boolean action(Event evt, Object arg) {
if (evt.target instanceof Button)
changeColor((String)arg);
return true;
}
void changeColor(String bname) {
if (bname.equals("Red")) setBackground(Color.red);
else if (bname.equals("Blue")) setBackground(Color.blue);
else if (bname.equals("Green")) setBackground(Color.green);
else if (bname.equals("White")) setBackground(Color.white);
else setBackground(Color.black);
}
}
Inheritance Example Color box in java
import java.awt.Graphics;
import java.awt.Color;
public class ColorBoxes extends java.applet.Applet {
public void paint(Graphics g) {
int rval, gval, bval;
for (int j = 30; j < (this.size().height -25); j += 30)
for (int i = 5; i < (this.size().width -25); i+= 30) {
rval = (int)Math.floor(Math.random() * 256);
gval = (int)Math.floor(Math.random() * 256);
bval = (int)Math.floor(Math.random() * 256);
g.setColor(new Color(rval,gval,bval));
g.fillRect(i,j,25,25);
g.setColor(Color.black);
g.drawRect(i-1,j-1,25,25);
}
}
}
public class ColorBoxes extends java.applet.Applet {
public void paint(Graphics g) {
int rval, gval, bval;
for (int j = 30; j < (this.size().height -25); j += 30)
for (int i = 5; i < (this.size().width -25); i+= 30) {
rval = (int)Math.floor(Math.random() * 256);
gval = (int)Math.floor(Math.random() * 256);
bval = (int)Math.floor(Math.random() * 256);
g.setColor(new Color(rval,gval,bval));
g.fillRect(i,j,25,25);
g.setColor(Color.black);
g.drawRect(i-1,j-1,25,25);
}
}
}
Program for Vowels And Consonants
public class VowelsAndConsonants {
public static void main(String[] args) {
for(int i = 0; i < 100; i++) {
char c = (char)(Math.random() * 26 + 'a');
System.out.print(c + ": ");
switch(c) {
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
System.out.println("vowel");
break;
case 'y':
case 'w':
System.out.println(
"Sometimes a vowel");
break;
default:
System.out.println("consonant");
}
}
}
}
public static void main(String[] args) {
for(int i = 0; i < 100; i++) {
char c = (char)(Math.random() * 26 + 'a');
System.out.print(c + ": ");
switch(c) {
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
System.out.println("vowel");
break;
case 'y':
case 'w':
System.out.println(
"Sometimes a vowel");
break;
default:
System.out.println("consonant");
}
}
}
}
Label in Java
import java.awt.*;
public class ColorTest extends java.applet.Applet {
ColorControls RGBcontrols, HSBcontrols;
Canvas swatch;
public void init() {
Color theColor = new Color(0,0,0);
float[] HSB = Color.RGBtoHSB(theColor.getRed(),
theColor.getGreen(), theColor.getBlue(),
(new float[3]));
setLayout(new GridLayout(1,3,10,10));
// The color swatch
swatch = new Canvas();
swatch.setBackground(theColor);
// the control panels
RGBcontrols = new ColorControls(this,"Red", "Green", "Blue",theColor.getRed(), theColor.getGreen(),theColor.getBlue());
HSBcontrols = new ColorControls(this,"Hue", "Saturation", "Brightness",(int)(HSB[0] * 360), (int)(HSB[1] * 100),(int)(HSB[2] * 100));
add(swatch);
add(RGBcontrols);
add(HSBcontrols);
}
public Insets insets() {
return new Insets(10,10,10,10);
}
void update(ColorControls in) {
Color c;
String v1 = in.f1.getText();
String v2 = in.f2.getText();
String v3 = in.f3.getText();
if (in == RGBcontrols) { // change to RGB
c = new Color(Integer.parseInt(v1),
Integer.parseInt(v2),
Integer.parseInt(v3));
swatch.setBackground(c);
float[] HSB = Color.RGBtoHSB(c.getRed(),c.getGreen(),
c.getBlue(), (new float[3]));
HSB[0] *= 360;
HSB[1] *= 100;
HSB[2] *= 100;
HSBcontrols.f1.setText(String.valueOf((int)HSB[0]));
HSBcontrols.f2.setText(String.valueOf((int)HSB[1]));
HSBcontrols.f3.setText(String.valueOf((int)HSB[2]));
}
else { // change to HSB
int f1 = Integer.parseInt(v1);
int f2 = Integer.parseInt(v2);
int f3 = Integer.parseInt(v3);
c = Color.getHSBColor((float)f1 / 360,
(float)f2 / 100, (float)f3/100);
swatch.setBackground(c);
RGBcontrols.f1.setText(String.valueOf(c.getRed()));
RGBcontrols.f2.setText(String.valueOf(
c.getGreen()));
RGBcontrols.f3.setText(String.valueOf(c.getBlue()));
}
}
}
class ColorControls extends Panel {
TextField f1, f2, f3;
ColorTest outerparent;
ColorControls(ColorTest target,
String l1, String l2, String l3,
int v1, int v2, int v3) {
this.outerparent = target;
setLayout(new GridLayout(3,4,10,10));
f1 = new TextField(String.valueOf(v1),10);
f2 = new TextField(String.valueOf(v2),10);
f3 = new TextField(String.valueOf(v3),10);
add(new Label(l1, Label.RIGHT));
add(f1);
add(new Label(l2, Label.RIGHT));
add(f2);
add(new Label(l3, Label.RIGHT));
add(f3);
}
public Insets insets() {
return new Insets(10,10,0,0);
}
public boolean action(Event evt, Object arg) {
if (evt.target instanceof TextField) {
this.outerparent.update(this);
// retrue true;
}
// else return false;
}
}
Swings in Java for a Labelpublic class ColorTest extends java.applet.Applet {
ColorControls RGBcontrols, HSBcontrols;
Canvas swatch;
public void init() {
Color theColor = new Color(0,0,0);
float[] HSB = Color.RGBtoHSB(theColor.getRed(),
theColor.getGreen(), theColor.getBlue(),
(new float[3]));
setLayout(new GridLayout(1,3,10,10));
// The color swatch
swatch = new Canvas();
swatch.setBackground(theColor);
// the control panels
RGBcontrols = new ColorControls(this,"Red", "Green", "Blue",theColor.getRed(), theColor.getGreen(),theColor.getBlue());
HSBcontrols = new ColorControls(this,"Hue", "Saturation", "Brightness",(int)(HSB[0] * 360), (int)(HSB[1] * 100),(int)(HSB[2] * 100));
add(swatch);
add(RGBcontrols);
add(HSBcontrols);
}
public Insets insets() {
return new Insets(10,10,10,10);
}
void update(ColorControls in) {
Color c;
String v1 = in.f1.getText();
String v2 = in.f2.getText();
String v3 = in.f3.getText();
if (in == RGBcontrols) { // change to RGB
c = new Color(Integer.parseInt(v1),
Integer.parseInt(v2),
Integer.parseInt(v3));
swatch.setBackground(c);
float[] HSB = Color.RGBtoHSB(c.getRed(),c.getGreen(),
c.getBlue(), (new float[3]));
HSB[0] *= 360;
HSB[1] *= 100;
HSB[2] *= 100;
HSBcontrols.f1.setText(String.valueOf((int)HSB[0]));
HSBcontrols.f2.setText(String.valueOf((int)HSB[1]));
HSBcontrols.f3.setText(String.valueOf((int)HSB[2]));
}
else { // change to HSB
int f1 = Integer.parseInt(v1);
int f2 = Integer.parseInt(v2);
int f3 = Integer.parseInt(v3);
c = Color.getHSBColor((float)f1 / 360,
(float)f2 / 100, (float)f3/100);
swatch.setBackground(c);
RGBcontrols.f1.setText(String.valueOf(c.getRed()));
RGBcontrols.f2.setText(String.valueOf(
c.getGreen()));
RGBcontrols.f3.setText(String.valueOf(c.getBlue()));
}
}
}
class ColorControls extends Panel {
TextField f1, f2, f3;
ColorTest outerparent;
ColorControls(ColorTest target,
String l1, String l2, String l3,
int v1, int v2, int v3) {
this.outerparent = target;
setLayout(new GridLayout(3,4,10,10));
f1 = new TextField(String.valueOf(v1),10);
f2 = new TextField(String.valueOf(v2),10);
f3 = new TextField(String.valueOf(v3),10);
add(new Label(l1, Label.RIGHT));
add(f1);
add(new Label(l2, Label.RIGHT));
add(f2);
add(new Label(l3, Label.RIGHT));
add(f3);
}
public Insets insets() {
return new Insets(10,10,0,0);
}
public boolean action(Event evt, Object arg) {
if (evt.target instanceof TextField) {
this.outerparent.update(this);
// retrue true;
}
// else return false;
}
}
import java.awt.*;
import javax.swing.*;
/* <applet code="JLabelDemo" width=355 height=200>
</applet>
*/
public class JLabelDemo extends JApplet{
public void init() {
// Get content pane
Container contentPane = getContentPane();
// Create an icon
ImageIcon ii = new ImageIcon("sateesh.jpg");
// Create a label
JLabel jl = new JLabel ("AVUL KALAM", ii, JLabel.CENTER);
// Add label to the content pane
contentPane.add(jl);
}
}
import javax.swing.*;
/* <applet code="JLabelDemo" width=355 height=200>
</applet>
*/
public class JLabelDemo extends JApplet{
public void init() {
// Get content pane
Container contentPane = getContentPane();
// Create an icon
ImageIcon ii = new ImageIcon("sateesh.jpg");
// Create a label
JLabel jl = new JLabel ("AVUL KALAM", ii, JLabel.CENTER);
// Add label to the content pane
contentPane.add(jl);
}
}
Simple Operators in Java Break And Continue
public class BreakAndContinue {
public static void main(String[] args) {
for(int i = 0; i < 100; i++) {
if(i == 74) break; // Out of for loop
if(i % 9 != 0) continue; // Next iteration
System.out.println(i);
}
int i = 0;
// An "infinite loop":
while(true) {
i++;
int j = i * 27;
if(j == 1269) break; // Out of loop
if(i % 10 != 0) continue; // Top of loop
System.out.println(i);
}
}
}
public static void main(String[] args) {
for(int i = 0; i < 100; i++) {
if(i == 74) break; // Out of for loop
if(i % 9 != 0) continue; // Next iteration
System.out.println(i);
}
int i = 0;
// An "infinite loop":
while(true) {
i++;
int j = i * 27;
if(j == 1269) break; // Out of loop
if(i % 10 != 0) continue; // Top of loop
System.out.println(i);
}
}
}
Conditional operator ?: (Ternary operator) in JAVA
class BigNo
{
public static void main (String args[])
{
int a = Integer.parseInt(args[0]);
int b = Integer.parseInt(args[1]);
int c = Integer.parseInt(args[2]);
int x = (a>b)?(a>c?a:c):(b>c?b:c);
System.out.println(+x);
}
}
{
public static void main (String args[])
{
int a = Integer.parseInt(args[0]);
int b = Integer.parseInt(args[1]);
int c = Integer.parseInt(args[2]);
int x = (a>b)?(a>c?a:c):(b>c?b:c);
System.out.println(+x);
}
}
How to Read values through(via) keyboard using Scanner
import java.util.*;
public class Array
{
public static void main(String
args[])
{
int[] a=new int[6];
Scanner sc=new
Scanner(System.in);
System.out.println("Please
enter elements...");
for(int j=0;j<6;j++)
a[j]=sc.nextInt();
System.out.println("Array
elements are : ");
for (int
i=0;i<a.length;i++)
System.out.println(a[i]);
}
}
Program on how to copy data from one array to another array
public class CopyArray {
public static void main(String[] args) {
int array1[]= {2,3,4,5,8,9};
int array2[] = new int[6];
System.out.println("array:");
System.out.print("[");
for (int i=0; i<array1.length; i++){
System.out.print(" "+array1[i]);
}
System.out.print("]");
System.out.println("\narray1:");
System.out.print("[");
for(int j=0; j<array1.length; j++){
array2[j] = array1[j];
System.out.print(" "+ array2[j]);
}
System.out.print("]");
}
}
public static void main(String[] args) {
int array1[]= {2,3,4,5,8,9};
int array2[] = new int[6];
System.out.println("array:");
System.out.print("[");
for (int i=0; i<array1.length; i++){
System.out.print(" "+array1[i]);
}
System.out.print("]");
System.out.println("\narray1:");
System.out.print("[");
for(int j=0; j<array1.length; j++){
array2[j] = array1[j];
System.out.print(" "+ array2[j]);
}
System.out.print("]");
}
}
Java program for an Array
import java.util.*;
public class ArrayDemo{
public static void main(String[] args){
int num[] = {50,20,45,82,25,63};
int l = num.length;
int i,j,t;
System.out.print("Given number : ");
for (i = 0;i < l;i++ ){
System.out.print(" " + num[i]);
}
System.out.println("\n");
System.out.print("Accending order number : ");
Arrays.sort(num);
for(i = 0;i < l;i++){
System.out.print(" " + num[i]);
}
}
}
public class ArrayDemo{
public static void main(String[] args){
int num[] = {50,20,45,82,25,63};
int l = num.length;
int i,j,t;
System.out.print("Given number : ");
for (i = 0;i < l;i++ ){
System.out.print(" " + num[i]);
}
System.out.println("\n");
System.out.print("Accending order number : ");
Arrays.sort(num);
for(i = 0;i < l;i++){
System.out.print(" " + num[i]);
}
}
}
JAVA Program for Constructor
class BoxDemo {
double width;
double height;
double depth;
// this is a constructor for Box
BoxDemo(){
System.out.println("Constructing BoxDemo");
width =10;
height =10;
depth =10;
}
//compute and return value
double volume(){
return width*height*depth;
}
}
class Box{
public static void main(String[] args) {
BoxDemo mybox = new BoxDemo();
double vol;
// get the volume of the box
vol = mybox.volume();
System.out.println("VOLUME IS "+vol);
}
}
// output : Constructing BoxDemo
// VOLUME IS 1000.0
double width;
double height;
double depth;
// this is a constructor for Box
BoxDemo(){
System.out.println("Constructing BoxDemo");
width =10;
height =10;
depth =10;
}
//compute and return value
double volume(){
return width*height*depth;
}
}
class Box{
public static void main(String[] args) {
BoxDemo mybox = new BoxDemo();
double vol;
// get the volume of the box
vol = mybox.volume();
System.out.println("VOLUME IS "+vol);
}
}
// output : Constructing BoxDemo
// VOLUME IS 1000.0
Constructor in JAVA (Parametrized)
class Box{double width;
double height;
double depth;
//this is the constructor for Box
Box(double w, double h, double d)
{
width = w;
height = h;
depth = d;
}
// compute and return value
double volume()
{
return width*height*depth;
}
}
class BoxParConst{
public static void main(String args[]){
// declare allocate and initialize Box objects
Box mybox1 = new Box(10,20,15);
Box mybox2 = new Box(3,6,9);
double vol;
// get the volume of first box
vol = mybox1.volume();
System.out.println("Volume of the first Box is:"+vol);
// get the volume of second box
vol = mybox2.volume();
System.out.println("Volume of the second Box is:"+vol);
}
}
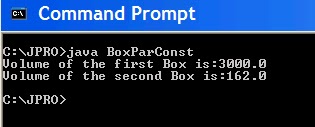
Program demo on How to Read a value through key board and print the factorial of a given number
import java.io.*;class Fact{
public static void main(String args[]){
BufferedReader Br = new BufferedReader(new InputStreamReader(System.in));
try{
int fact=1;
System.out.println("Enter a number to find out factorial of a given number: \n" );
String nu = Br.readLine();
int n = Integer.parseInt(nu);
for(int i=2;i<=n;i++)
{
fact=fact*i;
}
System.out.println("Factorial of a given number is:" +fact);
}catch (IOException err){
System.out.println("Error reading line");
}
}
}
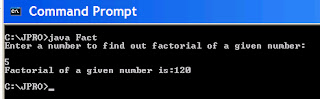
Program to convert a string from lower case to upper case
import java.lang.*;import java.io.*;
public class key
{
public static void main(String args[])throws IOException
{
try
{
BufferedReader br=new BufferedReader(new InputStreamReader(System.in));
System.out.println("enter the input from the key board");
String str=br.readLine();
System.out.println("Data enterd is "+str.toUpperCase());
}
catch(Exception e)
{
System.out.println(e);
}
}
}
Switch case simple program
class SwitchDemo{
public static void main(String args[])
{
int month=Integer.parseInt(args[0]);
switch(month)
{
case 1: System.out.println("January");break;
case 2: System.out.println("February");break;
case 3: System.out.println("March");break;
case 4: System.out.println("April");break;
case 5: System.out.println("May");break;
case 6: System.out.println("June");break;
case 7: System.out.println("July");break;
case 8: System.out.println("August");break;
case 9: System.out.println("September");break;
case 10: System.out.println("October"); break;
case 11: System.out.println("November"); break;
case 12: System.out.println("October"); break;
default: System.out.println("Sorry ! invallied choice");break;
}
}
}
JAVA Program for Combo Box using applet .
Attach the image part also using some image file
//JComboBox Demoimport java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/*
<applet code="JComboBoxDemo" width=300 height=100>
</applet>
*/
public class JComboBoxDemo extends JApplet
implements ItemListener {
JLabel jl;
ImageIcon Flowers, Koala, Desert, Penguins, sateesh;
public void init(){
// Get content pane
Container contentPane = getContentPane();
contentPane.setLayout(new FlowLayout());
// Create a Combo box and add it
//to the pane
JComboBox jc = new JComboBox();
jc.addItem("Flowers");
jc.addItem("Koala");
jc.addItem("Desert");
jc.addItem("Penguins");
jc.addItem("sateesh");
jc.addItemListener(this);
contentPane.add(jc);
//Create label
jl = new JLabel(new ImageIcon("Flowers.gif")); //attach image here
contentPane.add(jl);
}
public void itemStateChanged(ItemEvent ie) {
String s = (String)ie.getItem();
jl.setIcon(new ImageIcon(s +".gif"));
}
}
Program to create Check box in Java
This java program is used for displaying a clicked item in a text box
compiling: javac JCheckBoxDemo.java
Run: appletviewer JCheckBoxDemo.java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
/*
<applet code="JCheckBoxDemo" width=500 height=60>
</applet>
*/
public class JCheckBoxDemo extends JApplet
implements ItemListener {
JTextField jtf;
public void init(){
//Get content pane
Container contentPane = getContentPane();
contentPane.setLayout(new FlowLayout());
// Create icons
ImageIcon normal = new ImageIcon ("normal.gif");
ImageIcon rollover = new ImageIcon ("rollover.gif");
ImageIcon selected = new ImageIcon ("selected.gif");
// Add check boxes to the content pane
JCheckBox cb = new JCheckBox("C", normal);
cb.setRolloverIcon(rollover);
cb.setSelectedIcon(selected);
cb.addItemListener(this);
contentPane.add(cb);
cb = new JCheckBox("C++", normal);
cb.setRolloverIcon(rollover);
cb.setSelectedIcon(selected);
cb.addItemListener(this);
contentPane.add(cb);
cb = new JCheckBox("Java", normal);
cb.setRolloverIcon(rollover);
cb.setSelectedIcon(selected);
cb.addItemListener(this);
contentPane.add(cb);
cb = new JCheckBox("Perl", normal);
cb.setRolloverIcon(rollover);
cb.setSelectedIcon(selected);
cb.addItemListener(this);
contentPane.add(cb);
cb = new JCheckBox("VB", normal);
cb.setRolloverIcon(rollover);
cb.setSelectedIcon(selected);
cb.addItemListener(this);
contentPane.add(cb);
// Add text field to the content pane
jtf = new JTextField(15);
contentPane.add(jtf);
}
public void itemStateChanged(ItemEvent ie) {
JCheckBox cb = (JCheckBox)ie.getItem();
jtf.setText(cb.getText());
}
}
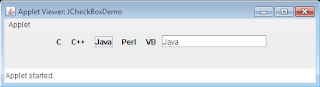
Java demo program for on Button using Applet
import java.awt.*;import java.awt.event.*;
import javax.swing.*;
/*
<applet code="JRadioButtonDemo" width=500 height=50>
</applet>
*/
public class JRadioButtonDemo extends JApplet
implements ActionListener {
JTextField jtf;
public void init(){
// Get content pane
Container contentPane = getContentPane();
contentPane.setLayout(new FlowLayout());
// ADD radio buttons to content pane
JRadioButton b1 = new JRadioButton("B.Sc");
b1.addActionListener(this);
contentPane.add(b1);
JRadioButton b2 = new JRadioButton("M.Sc");
b2.addActionListener(this);
contentPane.add(b2);
JRadioButton b3 = new JRadioButton("B.Tech");
b3.addActionListener(this);
contentPane.add(b3);
JRadioButton b4 = new JRadioButton("M.Tech");
b4.addActionListener(this);
contentPane.add(b4);
JRadioButton b5 = new JRadioButton("M.C.A");
b5.addActionListener(this);
contentPane.add(b5);
// Define a button group
ButtonGroup bg = new ButtonGroup();
bg.add(b1);
bg.add(b2);
bg.add(b3);
bg.add(b4);
bg.add(b5);
// Add text field to the content pane
jtf = new JTextField(15);
contentPane.add(jtf);
}
public void actionPerformed(ActionEvent ae){
jtf.setText(ae.getActionCommand());
}
}
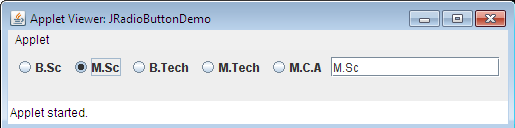
Java text field or you ca say a Text Box
compilation: javac JTextFieldDemo.javaRun: appletviewer JTextFieldDemo.java
import java.awt.*;
import javax.swing.*;
/* <applet code="JTextFieldDemo" width=200 height=50>
</applet>
*/
public class JTextFieldDemo extends JApplet{
public void init() {
// Get content pane
Container contentPane = getContentPane();
//Creating a Text filed
JTextField jtf = new JTextField(JTextField.CENTER);
// Add text field to content pane
contentPane.add(jtf);
}
}
Java program on Harmonic Series(HM)
class Harmonic{
public static void main (String args[])
{
int k,n=3;
double i=1,s,f=0;
while(i<=n)
{
s=1/i;
f=f+s;
i++;
}
for(k=1;k<=n;k++)
{
System.out.print("1/i"+k);
System.out.print(" ");
System.out.print(" + ");
System.out.print(" ");
}
System.out.print("-----------");
System.out.print("="+f);
}
}

Program for demo on Label and Image in Single code
This swing java program is used for displaying image, label in an applet.Place a required picture in a folder, copy and paste the following code in a note pad and
save as "JLabelDemo.java" as the file name. Note that picture and java file must be stored
in the same folder.
compilation: javac JLabelDemo.java
run: appletviewer JLabelDemo.java
import java.awt.*;
import javax.swing.*;
/* <applet code="JLabelDemo" width=355 height=200>
</applet>
*/
public class JLabelDemo extends JApplet{
public void init() {
// Get content pane
Container contentPane = getContentPane();
// Create an icon
ImageIcon ii = new ImageIcon("sateesh.jpg");
// Create a label
JLabel jl = new JLabel ("SATEESH.BAGADHI", ii, JLabel.CENTER);
// Add label to the content pane
contentPane.add(jl);
}
}
import javax.swing.*;
/* <applet code="JLabelDemo" width=355 height=200>
</applet>
*/
public class JLabelDemo extends JApplet{
public void init() {
// Get content pane
Container contentPane = getContentPane();
// Create an icon
ImageIcon ii = new ImageIcon("sateesh.jpg");
// Create a label
JLabel jl = new JLabel ("SATEESH.BAGADHI", ii, JLabel.CENTER);
// Add label to the content pane
contentPane.add(jl);
}
}
J applet for displaying a name
int no=10; // data members
void display(){ // method
System.out.println("Hai!");
}
}
class Main_Student{
public static void main(String args[]){
Student s = new Student(); //object s for Student class
System.out.println("Student number is:" +s.no);
s.display();
System.out.println("This is main class!");
}
}
import javax.swing.*;
/*
<applet code="JTabbedPaneDemo" width=400 height=100>
</applet>
*/
public class JTabbedPaneDemo extends JApplet {
public void init() {
JTabbedPane jtp = new JTabbedPane();
jtp.addTab("Cities", new CitiesPanel());
jtp.addTab("Colors", new ColorsPanel());
jtp.addTab("Flavors", new FlavorsPanel());
getContentPane().add(jtp);
}
}
class CitiesPanel extends JPanel {
public CitiesPanel() {
JButton b1 = new JButton("New York");
add(b1);
JButton b2 = new JButton("London");
add(b2);
JButton b3 = new JButton("Hong Kong");
add(b3);
JButton b4 = new JButton("Tokyo");
add(b4);
}
}
class ColorsPanel extends JPanel {
public ColorsPanel() {
JCheckBox cb1 = new JCheckBox("RED");
add(cb1);
JCheckBox cb2 = new JCheckBox("Green");
add(cb2);
JCheckBox cb3 = new JCheckBox("Blue");
add(cb3);
}
}
class FlavorsPanel extends JPanel {
public FlavorsPanel() {
JComboBox jcb = new JComboBox();
jcb.addItem("Vanilla");
jcb.addItem("Chocolate");
jcb.addItem("Strawberry");
add(jcb);
}
}
Compilation and Execution

import java.awt.Graphics;
import java.awt.Font;
import java.awt.Color;
public class MoreHelloApplet extends java.applet.Applet {
Font f = new Font("TimesRoman",Font.BOLD,36);
String name;
public void init() {
this.name = getParameter("name");
if (this.name == null)
this.name = "Avul Kalam";
this.name = "Hello " + this.name + "!";
}
public void paint(Graphics g) {
g.setFont(f);
g.setColor(Color.red);
g.drawString(this.name, 5, 50);
}
}
import java.awt.Font;
import java.awt.Color;
public class MoreHelloApplet extends java.applet.Applet {
Font f = new Font("TimesRoman",Font.BOLD,36);
String name;
public void init() {
this.name = getParameter("name");
if (this.name == null)
this.name = "Avul Kalam";
this.name = "Hello " + this.name + "!";
}
public void paint(Graphics g) {
g.setFont(f);
g.setColor(Color.red);
g.drawString(this.name, 5, 50);
}
}
Concept of Class Method and Object in JAVA
class Student{int no=10; // data members
void display(){ // method
System.out.println("Hai!");
}
}
class Main_Student{
public static void main(String args[]){
Student s = new Student(); //object s for Student class
System.out.println("Student number is:" +s.no);
s.display();
System.out.println("This is main class!");
}
}
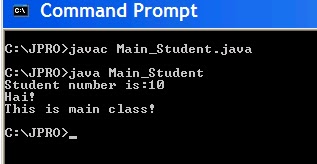
Java Program for Tabbed Pane in Java using applet
File name JTabbedPaneDemo.java
PROGRAMimport javax.swing.*;
/*
<applet code="JTabbedPaneDemo" width=400 height=100>
</applet>
*/
public class JTabbedPaneDemo extends JApplet {
public void init() {
JTabbedPane jtp = new JTabbedPane();
jtp.addTab("Cities", new CitiesPanel());
jtp.addTab("Colors", new ColorsPanel());
jtp.addTab("Flavors", new FlavorsPanel());
getContentPane().add(jtp);
}
}
class CitiesPanel extends JPanel {
public CitiesPanel() {
JButton b1 = new JButton("New York");
add(b1);
JButton b2 = new JButton("London");
add(b2);
JButton b3 = new JButton("Hong Kong");
add(b3);
JButton b4 = new JButton("Tokyo");
add(b4);
}
}
class ColorsPanel extends JPanel {
public ColorsPanel() {
JCheckBox cb1 = new JCheckBox("RED");
add(cb1);
JCheckBox cb2 = new JCheckBox("Green");
add(cb2);
JCheckBox cb3 = new JCheckBox("Blue");
add(cb3);
}
}
class FlavorsPanel extends JPanel {
public FlavorsPanel() {
JComboBox jcb = new JComboBox();
jcb.addItem("Vanilla");
jcb.addItem("Chocolate");
jcb.addItem("Strawberry");
add(jcb);
}
}
Compilation and Execution
Java program demo on tool tip
//Java Core Packageimport javax.swing.*;
//Java Extension Package
import java.awt.*;
public class JavaApplication2 extends JFrame {
//Initializing JButton
private JButton button;
//Setting up GUI
public JavaApplication2() {
//Setting up the Title of the Window
super("Add ToolTipText on JButton");
//Set Size of the Window (WIDTH, HEIGHT)
setSize(250,100);
//Exit Property of the Window
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//Constructing JButton
button = new JButton("Place The Cursor Here...");
//Setting or Adding ToolTipText on JButton
button.setToolTipText("My First ToolTipText on JButton");
//Setting up the container ready for the components to be added.
Container pane = getContentPane();
setContentPane(pane);
//Setting up the container layout
FlowLayout flow = new FlowLayout(FlowLayout.CENTER);
pane.setLayout(flow);
//Adding the JButton component to the container
pane.add(button);
/**Set all the Components Visible.
* If it is set to "false", the components in the container will not be visible.
*/
setVisible(true);
}
//Main Method
public static void main (String[] args) {
JavaApplication2 ttt = new JavaApplication2();
}
}
JAVA IO for displaying student details using fopen flcose
import java.io.*;
class Student{
public static void main(String args[]){
InputStreamReader is = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(is);
System.out.print("Welcome to java");
try{
System.out.println("Enter Student Name:");
String Name = br.readLine();
System.out.println("Enter Student Number:");
String No = br.readLine();
System.out.println("Enter Student class:");
String Clas = br.readLine();
System.out.println(" Hi "+Name +" your no is: "+ No +", you are studying "+Clas +"!");
}
catch (IOException Err) {
System.out.print("Error in reading Line!");
}
catch(NumberFormatException Err){
System.out.print("Error in Number format conversion!");
}
}
}
class Student{
public static void main(String args[]){
InputStreamReader is = new InputStreamReader(System.in);
BufferedReader br = new BufferedReader(is);
System.out.print("Welcome to java");
try{
System.out.println("Enter Student Name:");
String Name = br.readLine();
System.out.println("Enter Student Number:");
String No = br.readLine();
System.out.println("Enter Student class:");
String Clas = br.readLine();
System.out.println(" Hi "+Name +" your no is: "+ No +", you are studying "+Clas +"!");
}
catch (IOException Err) {
System.out.print("Error in reading Line!");
}
catch(NumberFormatException Err){
System.out.print("Error in Number format conversion!");
}
}
}
Jdbc(Java Database Connectivity) Odbc(Open Database Conectiy) program using MS-Access database (4 Drivers)
import javax.swing.*;
import java.awt.event.*;
import java.sql.*;
import java.awt.*;
class Myframe extends JFrame implements ActionListener
{
JLabel lno,lname;
JTextField tno,tname;
JButton insert,view,update,search,nxt,pre,lst,frt,clr,dlt,exit;
Connection cn;
Statement st;
ResultSet rs;
Container conpane;
Myframe()
{
lno=new JLabel("Eno");
lname=new JLabel("Ename");
tno=new JTextField(6);
tname=new JTextField(15);
insert=new JButton("Insert");
view=new JButton("View");
nxt=new JButton("Next");
pre=new JButton("Previous");
lst=new JButton("Last");
frt=new JButton("First");
clr=new JButton("Clear");
dlt=new JButton("Delete");
update=new JButton("Update");
search=new JButton("Search");
exit=new JButton("Exit");
conpane = getContentPane();
JPanel txtPanel,btnPanel;
txtPanel = new JPanel();
btnPanel = new JPanel();
//setLayout(new GridLayout(2,2));
conpane.add(txtPanel,BorderLayout.NORTH);
conpane.add(btnPanel,BorderLayout.CENTER);
txtPanel.add(lno);
txtPanel.add(tno);
txtPanel.add(lname);
txtPanel.add(tname);
btnPanel.add(insert);
btnPanel.add(view);
btnPanel.add(nxt);
btnPanel.add(pre);
btnPanel.add(lst);
btnPanel.add(frt);
btnPanel.add(clr);
btnPanel.add(dlt);
btnPanel.add(update);
btnPanel.add(search);
btnPanel.add(exit);
insert.addActionListener(this);
view.addActionListener(this);
nxt.addActionListener(this);
pre.addActionListener(this);
lst.addActionListener(this);
frt.addActionListener(this);
clr.addActionListener(this);
dlt.addActionListener(this);
update.addActionListener(this);
search.addActionListener(this);
exit.addActionListener(this);
try
{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
cn=DriverManager.getConnection("jdbc:odbc:employeedsn");
st=cn.createStatement(ResultSet.TYPE_SCROLL_SENSITIVE,ResultSet.CONCUR_UPDATABLE);
rs=st.executeQuery("select * from emp");
rs.next();
}catch(Exception e){}
}
public void actionPerformed(ActionEvent a)
{
try
{
if(a.getSource()==search)
{
dbOpen();
String str = JOptionPane.showInputDialog(null, "Enter empno : ");
rs=st.executeQuery("select * from emp where eno="+str+"");
rs.next();
setText();
}
if(a.getSource()==update)
{
dbOpen();
int ueno=Integer.parseInt(tno.getText());
String uname=(tname.getText());
st.executeUpdate("UPDATE emp SET eno=" + ueno +",ename='" + tname.getText() + "' WHERE eno="+ ueno +" ");
JOptionPane.showMessageDialog(null,"Record is updated");
}
if(a.getSource()==dlt)
{
int deno=Integer.parseInt(tno.getText());
st.executeUpdate("DELETE FROM emp WHERE eno="+ deno);
JOptionPane.showMessageDialog(null,"Record is deleted");
dbClose();
dbOpen();
}
if(a.getSource()==clr)
{
tno.setText("");
tname.setText("");
rs.first();
}
if(a.getSource()==frt)
{
rs.first();
setText();
}
if(a.getSource()==lst)
{
rs.last();
setText();
}
if(a.getSource()==nxt)
{
if(!rs.isAfterLast())
{
rs.next();
setText();
}
else
{
JOptionPane.showMessageDialog(null,"Last Record");
rs.previous();
}
}
if(a.getSource()==pre)
{
if(!rs.isBeforeFirst())
{
rs.previous();
setText();
}
else
{
JOptionPane.showMessageDialog(null,"First Record");
rs.first();
}
}
if(a.getSource()==insert)
{
int eno=Integer.parseInt(tno.getText());
String name=(tname.getText());
st.executeUpdate("insert into emp values("+eno+",'"+name+"')");
JOptionPane.showMessageDialog(null,"Record inserted into database");
dbClose();
dbOpen();
}
if(a.getSource()==view)
{
rs.next();
setText();
dbClose();
dbOpen();
}
if(a.getSource()==exit)
{
if(JOptionPane.showConfirmDialog(null,"Are You Sure You Want to Exit?","Confirm",JOptionPane.YES_NO_OPTION)==JOptionPane.YES_OPTION)
System.exit(0);
}
}catch(Exception e)
{
}
}
public void dbOpen()
{
try
{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
cn=DriverManager.getConnection("jdbc:odbc:employeedsn");
st=cn.createStatement(ResultSet.TYPE_SCROLL_SENSITIVE,ResultSet.CONCUR_UPDATABLE);
rs=st.executeQuery("select * from emp");
rs.next();
}catch(Exception e){}
}
public void setText(){
try{
tno.setText(rs.getString(1));
tname.setText(rs.getString(2));
}catch(Exception ex){}
}
public void dbClose()
{
try{
st.close();
rs.close();
cn.close();
}catch(Exception e){}
}
}
class jdbcdemoframe
{
public static void main(String s[])
{
Myframe f= new Myframe();
f.setVisible(true);
f.setTitle("Employee Information");
f.setSize(350,250);
}
}
import java.awt.event.*;
import java.sql.*;
import java.awt.*;
class Myframe extends JFrame implements ActionListener
{
JLabel lno,lname;
JTextField tno,tname;
JButton insert,view,update,search,nxt,pre,lst,frt,clr,dlt,exit;
Connection cn;
Statement st;
ResultSet rs;
Container conpane;
Myframe()
{
lno=new JLabel("Eno");
lname=new JLabel("Ename");
tno=new JTextField(6);
tname=new JTextField(15);
insert=new JButton("Insert");
view=new JButton("View");
nxt=new JButton("Next");
pre=new JButton("Previous");
lst=new JButton("Last");
frt=new JButton("First");
clr=new JButton("Clear");
dlt=new JButton("Delete");
update=new JButton("Update");
search=new JButton("Search");
exit=new JButton("Exit");
conpane = getContentPane();
JPanel txtPanel,btnPanel;
txtPanel = new JPanel();
btnPanel = new JPanel();
//setLayout(new GridLayout(2,2));
conpane.add(txtPanel,BorderLayout.NORTH);
conpane.add(btnPanel,BorderLayout.CENTER);
txtPanel.add(lno);
txtPanel.add(tno);
txtPanel.add(lname);
txtPanel.add(tname);
btnPanel.add(insert);
btnPanel.add(view);
btnPanel.add(nxt);
btnPanel.add(pre);
btnPanel.add(lst);
btnPanel.add(frt);
btnPanel.add(clr);
btnPanel.add(dlt);
btnPanel.add(update);
btnPanel.add(search);
btnPanel.add(exit);
insert.addActionListener(this);
view.addActionListener(this);
nxt.addActionListener(this);
pre.addActionListener(this);
lst.addActionListener(this);
frt.addActionListener(this);
clr.addActionListener(this);
dlt.addActionListener(this);
update.addActionListener(this);
search.addActionListener(this);
exit.addActionListener(this);
try
{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
cn=DriverManager.getConnection("jdbc:odbc:employeedsn");
st=cn.createStatement(ResultSet.TYPE_SCROLL_SENSITIVE,ResultSet.CONCUR_UPDATABLE);
rs=st.executeQuery("select * from emp");
rs.next();
}catch(Exception e){}
}
public void actionPerformed(ActionEvent a)
{
try
{
if(a.getSource()==search)
{
dbOpen();
String str = JOptionPane.showInputDialog(null, "Enter empno : ");
rs=st.executeQuery("select * from emp where eno="+str+"");
rs.next();
setText();
}
if(a.getSource()==update)
{
dbOpen();
int ueno=Integer.parseInt(tno.getText());
String uname=(tname.getText());
st.executeUpdate("UPDATE emp SET eno=" + ueno +",ename='" + tname.getText() + "' WHERE eno="+ ueno +" ");
JOptionPane.showMessageDialog(null,"Record is updated");
}
if(a.getSource()==dlt)
{
int deno=Integer.parseInt(tno.getText());
st.executeUpdate("DELETE FROM emp WHERE eno="+ deno);
JOptionPane.showMessageDialog(null,"Record is deleted");
dbClose();
dbOpen();
}
if(a.getSource()==clr)
{
tno.setText("");
tname.setText("");
rs.first();
}
if(a.getSource()==frt)
{
rs.first();
setText();
}
if(a.getSource()==lst)
{
rs.last();
setText();
}
if(a.getSource()==nxt)
{
if(!rs.isAfterLast())
{
rs.next();
setText();
}
else
{
JOptionPane.showMessageDialog(null,"Last Record");
rs.previous();
}
}
if(a.getSource()==pre)
{
if(!rs.isBeforeFirst())
{
rs.previous();
setText();
}
else
{
JOptionPane.showMessageDialog(null,"First Record");
rs.first();
}
}
if(a.getSource()==insert)
{
int eno=Integer.parseInt(tno.getText());
String name=(tname.getText());
st.executeUpdate("insert into emp values("+eno+",'"+name+"')");
JOptionPane.showMessageDialog(null,"Record inserted into database");
dbClose();
dbOpen();
}
if(a.getSource()==view)
{
rs.next();
setText();
dbClose();
dbOpen();
}
if(a.getSource()==exit)
{
if(JOptionPane.showConfirmDialog(null,"Are You Sure You Want to Exit?","Confirm",JOptionPane.YES_NO_OPTION)==JOptionPane.YES_OPTION)
System.exit(0);
}
}catch(Exception e)
{
}
}
public void dbOpen()
{
try
{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
cn=DriverManager.getConnection("jdbc:odbc:employeedsn");
st=cn.createStatement(ResultSet.TYPE_SCROLL_SENSITIVE,ResultSet.CONCUR_UPDATABLE);
rs=st.executeQuery("select * from emp");
rs.next();
}catch(Exception e){}
}
public void setText(){
try{
tno.setText(rs.getString(1));
tname.setText(rs.getString(2));
}catch(Exception ex){}
}
public void dbClose()
{
try{
st.close();
rs.close();
cn.close();
}catch(Exception e){}
}
}
class jdbcdemoframe
{
public static void main(String s[])
{
Myframe f= new Myframe();
f.setVisible(true);
f.setTitle("Employee Information");
f.setSize(350,250);
}
}
DATA STRUCTURES
STACK follows LIFO of FILO Last In First Out or First In Last Out
Operations PUSH(Add) POP (Delete)
class Stack {
int[] a;
int top;
public Stack(int n) {
a = new int[n];
top = -1;
}
public void push(int val) {
if (top == a.length - 1) {
System.out.println("Stack overflow...");
} else {
top++;
a[top] = val;
}
}
public void pop() {
if (top == -1) {
System.out.println("Stack underflow...");
} else {
System.out.println("Element popped" + a[top]);
top--;
}
}
public void display() {
if (top == -1) {
System.out.println("Stack empty...");
} else {
for (int i = top; i >= 0; i--) {
System.out.println("Stack element: " + a[i]);
}
}
}
}
public class UseStack {
static public void main(String args[]) {
Scanner sc = new Scanner(System.in);
Stack s;
int n;
System.out.println("Enter the size of the stack: ");
n = sc.nextInt();
s = new Stack(n);
int choice;
do {
System.out.println("1.PUSH" + "\n" + "2.POP" + "\n" + "3.DISPLAY" + "\n" + "0.EXIT");
System.out.println("Enter your choice");
choice = sc.nextInt();
switch (choice) {
case 1:
int value;
System.out.println("Enter element to push");
value = sc.nextInt();
s.push(value);
break;
case 2:
s.pop();
break;
case 3:
s.display();
case 0:
break;
default:
System.out.println("invalid choice...");
}
} while (choice != 0);
}
}
class City
{
public static void main(String args[])
{
String name[] = {"visakhapatnam","hyderabad","kolkata","mumbai","chennai","delhi","ahamdabad","calcutta","bombay"};
int size=name.length;
String temp;
System.out.println("The total number of the strings are:" + name.length);
for(int i=0;i<size;i++)
{
for(int j=i+1;j<size;j++)
{
if(name[j].compareTo(name[i])<0)
{
temp=name[i];
name[i]=name[j];
name[j]=temp;
}
}
}
for(int i=0;i<size;i++)
{
System.out.println(name[i]);
}
}
}
STACK Program using JAVA
STACK follows LIFO of FILO Last In First Out or First In Last Out
Operations PUSH(Add) POP (Delete)
import java.util.Scanner;
class Stack {
int[] a;
int top;
public Stack(int n) {
a = new int[n];
top = -1;
}
public void push(int val) {
if (top == a.length - 1) {
System.out.println("Stack overflow...");
} else {
top++;
a[top] = val;
}
}
public void pop() {
if (top == -1) {
System.out.println("Stack underflow...");
} else {
System.out.println("Element popped" + a[top]);
top--;
}
}
public void display() {
if (top == -1) {
System.out.println("Stack empty...");
} else {
for (int i = top; i >= 0; i--) {
System.out.println("Stack element: " + a[i]);
}
}
}
}
public class UseStack {
static public void main(String args[]) {
Scanner sc = new Scanner(System.in);
Stack s;
int n;
System.out.println("Enter the size of the stack: ");
n = sc.nextInt();
s = new Stack(n);
int choice;
do {
System.out.println("1.PUSH" + "\n" + "2.POP" + "\n" + "3.DISPLAY" + "\n" + "0.EXIT");
System.out.println("Enter your choice");
choice = sc.nextInt();
switch (choice) {
case 1:
int value;
System.out.println("Enter element to push");
value = sc.nextInt();
s.push(value);
break;
case 2:
s.pop();
break;
case 3:
s.display();
case 0:
break;
default:
System.out.println("invalid choice...");
}
} while (choice != 0);
}
}
Program in JAVA for string Sorting
import java.lang.*;class City
{
public static void main(String args[])
{
String name[] = {"visakhapatnam","hyderabad","kolkata","mumbai","chennai","delhi","ahamdabad","calcutta","bombay"};
int size=name.length;
String temp;
System.out.println("The total number of the strings are:" + name.length);
for(int i=0;i<size;i++)
{
for(int j=i+1;j<size;j++)
{
if(name[j].compareTo(name[i])<0)
{
temp=name[i];
name[i]=name[j];
name[j]=temp;
}
}
}
for(int i=0;i<size;i++)
{
System.out.println(name[i]);
}
}
}
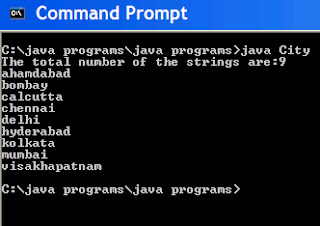